VB.NETでは繰り返し文が複数用意されています。
- While 条件式 ~ End While
- Do While 条件式 Loop
- Do ~ Loop While 条件式
- Do Until 条件式 ~ Loop(←本記事)
- Do ~ Loop Until 条件式
繰り返し処理の種類が色々あって「使い方がわからん!」と思う方が多いかもしれませんが、落ち着いて考えれば「なるほど」と思えます。今回は「Do Until 条件式 ~ Loop」の使い方を解説します。
Do Until 条件式 Loop
MSDNを見てみると、「Do Until 条件式 Loopなんてページ無いじゃん!」と思います。仕方ありません。個別のページを設けるということを行わない代償として、総合的に見れるページが用意されています。ただし、理解するのに時間がかかります。そんなことをしていると進捗状況に遅延が発生します。

ページの先頭に、「Boolean 条件が True の場合、または条件が True になるまで、ステートメントのブロックを繰り返します。」と書いてあります。よくわかりませんね。まずは下記の構文を見て下さい。
Do Until 条件式 繰り返す処理 Loop
Do Until~ Loop は、「条件式がFalseの間はループを続ける」という特徴があります。Do While ~ Loop と逆ですね(Do While ~ Loopは条件式がTrueの間はループを続けます)。
尚、無限ループを作りたい場合は以下の様にすれば作れますが、基本的にはやらないでください。
Do While 1 <= 0 繰り返す処理 Loop
もし間違ってデバッグで上記を実行した場合は強制終了、exeを実行して無限ループが発生した場合はタスクマネージャーから強制終了するなどしてプログラム実行を止めて下さい(くれぐれも無限ループはやらないでね)。
使い方例
Do Until~ Loop の実行確認をするために、サンプルのフォームを作ってみました。
開始値と終了値を入力し、実行ボタンを押すと、終了値までに1つずつ値を減らし、減った分だけ文字列を結合するという検証用のフォームです。例えば、
- 開始値:5
- 終了値:1
という場合、実行ボタンを押すと、結果が54321になります。サンプルソースはこんな感じです。
Private Sub cmdDoUntilLoop_Click(sender As Object, e As EventArgs) Handles cmdDoUntilLoop.Click Try 'テキストボックスに入力された値をInteger型の変数に代入 Dim intStartValue As Integer = Integer.Parse(txtStartValue.Text) Dim intEndValue As Integer = Integer.Parse(txtEndValue.Text) Dim strResult As String = Nothing '開始値が終了値より小さくなるまで繰り返し Do Until intStartValue < intEndValue strResult = strResult & intStartValue.ToString intStartValue = intStartValue - 1 Loop MessageBox.Show(strResult, "結果", MessageBoxButtons.OK, MessageBoxIcon.Information) txtResult.Text = strResult Catch ex As Exception MessageBox.Show(ex.Message) End Try End Sub
Do Until~ Loop の部分です。開始値が終了値と同じになるまでループを繰り返します。
'開始値が終了値より小さくなるまで繰り返し Do Until intStartValue < intEndValue strResult = strResult & intStartValue.ToString intStartValue = intStartValue - 1 Loop
ループ内の処理は「文字列の結合」と「intStartValueの減算」です。実行してみましょう。
開始値に5、終了値に1を入力し、実行ボタンを押下します。
はい、順番に5から1の数字が並んで出てきました。最後に結果テキストボックスに入っていればOKです。
ちゃんと出てきましたね。
Do Until~ Loop の動きを見てみます。
【開始前】
開始値:5
終了値:1
結果出力用文字列:Nothing【1週目】
intStartValue:5
intEndValue:1
条件 intStartValue < intEndValue を満たさないのでDo Untilの中に入る
strResult に 5 を入れる(代入後のstrResult の中身:5)
intStartValue を1減らす【2週目】
intStartValue:4
intEndValue:1
条件 intStartValue < intEndValue を満たさないのでDo Untilの中に入る
strResult に 4 を入れる(代入後のstrResult の中身:54)
intStartValue を1減らす【3週目】
intStartValue:3
intEndValue:1
条件 intStartValue < intEndValue を満たさないのでDo Untilの中に入る
strResult に 3 を入れる(代入後のstrResult の中身:543)
intStartValue を1減らす【4週目】
intStartValue:2
intEndValue:1
条件 intStartValue < intEndValue を満たさないのでDo Untilの中に入る
strResult に 2 を入れる(代入後のstrResult の中身:5432)
intStartValue を1減らす【5週目】
intStartValue:1
intEndValue:1
条件 intStartValue < intEndValue を満たさないのでDo Untilの中に入る
strResult に 1 を入れる(代入後のstrResult の中身:54321)
intStartValue を1減らす【6週目】
intStartValue:0
intEndValue:1
条件 intStartValue < intEndValue を満たすのでDo Untilの中に入らない
5週目のループの中でintStartValueの値が0になり、6週目のDo Until開始前の条件判定で、条件式が intStartValue > intEndValue となったため、6週目のループには入りません。
オマケ:事前チェック入れてみる
ここはオマケなのですが、今回、サンプルで作成したフォームにチェック処理を入れてません。未入力や文字列を入れたら落ちます。なので、チェック処理を入れると以下のソースとなります。
Private Sub cmdDoUntilLoop_Click(sender As Object, e As EventArgs) Handles cmdDoUntilLoop.Click Try '事前チェック If String.IsNullOrEmpty(txtStartValue.Text) = True Then MessageBox.Show("開始値を入力してください!", "入力チェック", MessageBoxButtons.OK, MessageBoxIcon.Exclamation) Exit Try ElseIf String.IsNullOrEmpty(txtEndValue.Text) = True Then MessageBox.Show("終了値を入力してください!", "入力チェック", MessageBoxButtons.OK, MessageBoxIcon.Exclamation) Exit Try End If If IsNumeric(txtStartValue.Text) = False Then MessageBox.Show("開始値には数字を入力してください!", "入力チェック", MessageBoxButtons.OK, MessageBoxIcon.Exclamation) Exit Try ElseIf IsNumeric(txtEndValue.Text) = False Then MessageBox.Show("終了値には数字を入力してください!", "入力チェック", MessageBoxButtons.OK, MessageBoxIcon.Exclamation) Exit Try End If 'テキストボックスに入力された値をInteger型の変数に代入 Dim intStartValue As Integer = Integer.Parse(txtStartValue.Text) Dim intEndValue As Integer = Integer.Parse(txtEndValue.Text) Dim strResult As String = Nothing '開始値が終了値とより小さくなるまで繰り返し Do Until intStartValue < intEndValue strResult = strResult & intStartValue.ToString intStartValue = intStartValue - 1 Loop MessageBox.Show(strResult, "結果", MessageBoxButtons.OK, MessageBoxIcon.Information) txtResult.Text = strResult Catch ex As Exception MessageBox.Show(ex.Message) End Try End Sub
事前チェックに2種類のチェックを入れています。未入力チェックと入力値の数値チェックです。
編集後記
実はちらほら見かける「Do Until~ Loop」なので、覚えておいた方が良いですね。「条件を満たさない間は続ける」というのも違和感があり、慣れていないとちょっと分かりにくいかもしれませんが、基本的には「Do While ~ Loop」と同じ動きをするので、「Do While ~ Loop」を理解していれば、「Do Until~ Loop」もすんなりと理解出来ると思います。
関連記事
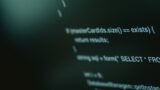
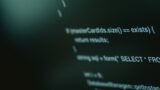
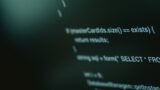
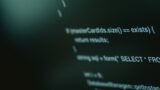
参考サイト

.